Addressing some of the technical debt. Not very exciting. So bullet lists.
DID:
- Set up Jasmine tests in Robotron (8 specs, 0 failures)
- Set up Jasmine tests in
canvas-libs
(4 specs, 0 failures) - Moved
supporting.js
stuff tocanvas-libs
for both Centipede and Robotron - Moved the Sound object from
sound.js
tocanvas-libs
for both Centipede and Robotron
Commits
Centipede
Robotron
Canvas Libs
Still TODO:
- Add more tests to Robotron
- Add more tests to
canvas-libs
- Add tests to Joust
- Fix broken tests in Centipede. All of the refactoring has resulted in 59 specs, 37 failures :/
- Research whether it’s possible to run Jasmine tests with a pipeline script in GitLab, so the results can be seen in the repo
The test spec runners
Spec runners are accessible through eash app’s URL, but the latest code is not yet deployed:
Not canvas libs, though! Maybe I’ll add those later.
Local results:
Centipede
Robotron
Notes
I’ll need to come up with a logical naming scheme for overrides of things pulled from canvas-libs
. Can I just name it the same thing? Or should I prepend the project name to it, like var robotronControls = controls;
?
Init functions
I had this great idea to put all of the initialization scripts inside game.start()
.
This worked to run the game, but created problems in testing, where any game.start()
call would generate a ton of DOM elements, which I’m not really sure I want to be doing during the tests. I could probably block creation of the elements somehow, or find another way to test the start script without actually running it.
The workaround for now is to just move all the init function calls to an init.js
script, and load that last, and to call that out explicitly in the index.html
so I don’t muck it up later.
|
|
Yawn, where’s the game stuff
Sorry not sorry. This stuff is important. With tests and libraries in place, I can break up tight coupling so the games are easy to understand and easily extensible. This way I’ll know exactly where that thing I just changed will impact the entire game without having to trace logic.
March 16, 2018 Update
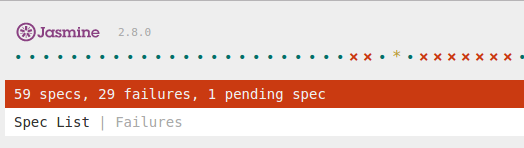
Commits
- 3251fd5 get 3 tests passing [Matthew Odle]
- 36c1612 get more tests passing [Matthew Odle]
- 889f7ec all interval-creatures-spec tests are passing [Matthew Odle]
Several more interval-creatures-spec.js
tests are passing. The primary issue causing these tests to fail previously was around the restructuring of the manage
function. Much of this was offloaded to subfunctions in the refactor, so these new subfunctions had to be spied on.
The refactored manage function:
|
|
The setup mock function:
|
|
The test, before:
|
|
The error (it’s complaining about intervalCreatures.intervals
being undefined
, because that gets set in intervalCreatures.init()
, which never gets called. First, I executed init()
, but eventually opted just to set dummy data in the interval
property):
|
|
The test, after:
|
|
The default objects had to be set in the beforeEach
stage to avoid undefined reference errors.
Object initialization (using Object.assign
) was also moved into beforeEach
, to ensure a fresh copy of the object is being used for each test.
|
|
This highlighted a problem in interval-creatures.js
, where a static reference was being used instead of an instance reference, causing the creature arrays (flies
, worms
) to be empty, even after adding a value to the array during the test setup, with testObj.intervals['worms'] = 10;
. And the empty creature arrays passed the intervalCreatures[creature] == false
test, so manage
never got to clearOutsideCanvas
.
Before:
|
|
After:
|
|